Hi Walter,
I have a different question related to segmentIndex
. In the diagram below, the right arm (from Alpha to Gamma) is a custom link with its link.points
specified
const pts = link.points.copy();
pts.clear();
pts.add(new go.Point(200, 125));
pts.add(new go.Point(350, 125));
pts.add(new go.Point(350, 200));
link.points = pts;
The left arm and the central vertical link are automatically routed by GoJS.
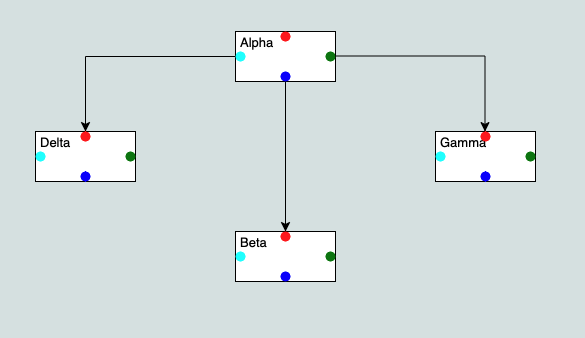
When I manipulated the segmentIndex
value in the link template, I observed some issues that I do not quite understand.
myDiagram.linkTemplate = $(
go.Link,
{ routing: go.Link.Orthogonal },
$(go.Shape),
$(go.Shape, { toArrow: "Standard" }),
$(go.TextBlock, "label", { segmentIndex: NaN, segmentFraction: 0.5 })
);
When I am using NaN
as the value, the label is centered at all the links, which makes sense.
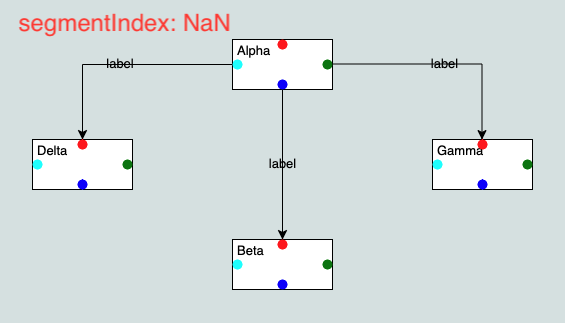
When I change the segmentIndex
value to 0, the label’s position in the right arm link makes sense to me because it is centered at the first segment. But why the labels in the other 2 links are positioned at the fromPort?
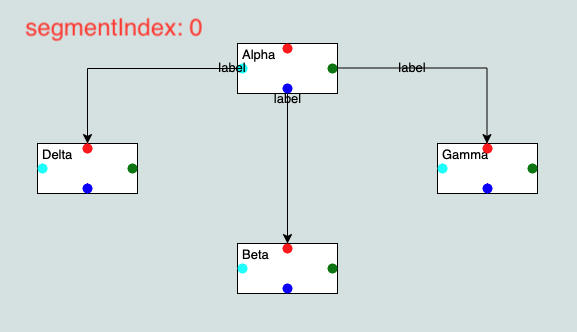
When I change the segmentIndex
value to 1, the label’s position in the right arm link makes sense to me too because it is centered at the second segment. But again, I do not understand how the labels in the other 2 links are positioned.
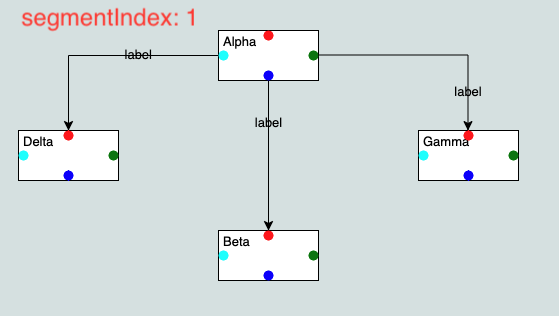
For more references, the figures below show the label positions with segmentIndex
to be 2, 3, and 4.
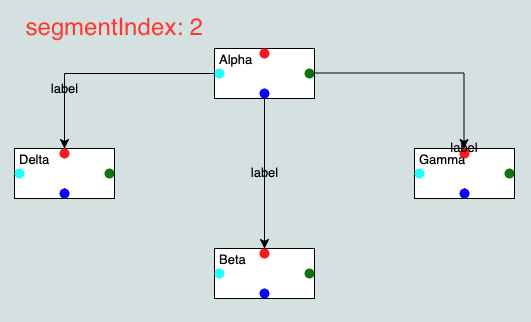
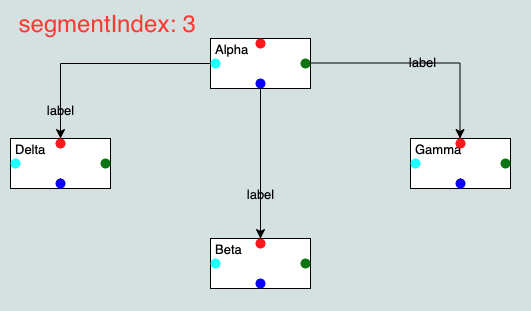
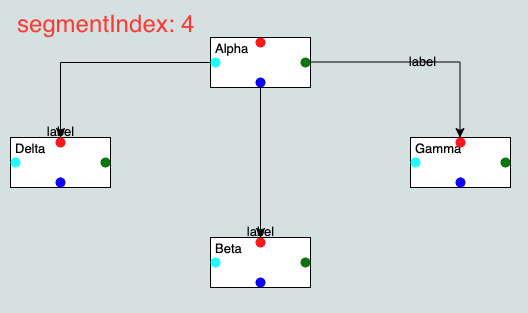
How does GoJS count segments for automatically routed links? When an automatically routed link that has a horizontal part and a vertical part, that is, the left arm from Alpha to Delta, how can I make sure the label is positioned center in the vertical part?
I am pasting my HTML + JS code below. I am using NaN
as the default segmentIndex
value. But you could tweak that value if you want.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div
id="myDiagramDiv"
style="width: 1500px; height: 800px; background-color: #dae4e4"
></div>
<script src="../../release/go-debug.js"></script>
<script>
function TestLayout() {
go.Layout.call(this);
}
go.Diagram.inherit(TestLayout, go.Layout);
TestLayout.prototype.doLayout = function () {
let alpha;
let beta;
let gamma;
let delta;
const it = this.diagram.nodes.iterator;
while (it.next()) {
const node = it.value;
if (node.key === "Alpha") {
alpha = node;
} else if (node.key === "Beta") {
beta = node;
} else if (node.key === "Gamma") {
gamma = node;
} else if (node.key === "Delta") {
delta = node;
}
}
this.diagram.startTransaction("Test Layout");
alpha.moveTo(100, 100);
beta.moveTo(100, 300);
gamma.moveTo(300, 200);
delta.moveTo(-100, 200);
const linkIt = alpha.findLinksOutOf();
while (linkIt.next()) {
const link = linkIt.value;
if (link.toNode.key === "Gamma") {
const pts = link.points.copy();
pts.clear();
pts.add(new go.Point(200, 125));
pts.add(new go.Point(350, 125));
pts.add(new go.Point(350, 200));
link.points = pts;
}
}
this.diagram.commitTransaction("Test Layout");
};
const $ = go.GraphObject.make;
const myDiagram = $(go.Diagram, "myDiagramDiv", {
layout: $(TestLayout),
});
myDiagram.nodeTemplate = $(
go.Node,
"Position",
$(go.Shape, "Rectangle", {
width: 100,
height: 50,
fill: "white",
}),
$(go.TextBlock, { margin: 5 }, new go.Binding("text", "key")),
// ports
$(go.Shape, "Circle", {
portId: "input",
width: 10,
height: 10,
fill: "red",
stroke: null,
position: new go.Point(45, 0),
toSpot: go.Spot.Top,
}),
$(go.Shape, "Circle", {
portId: "output",
width: 10,
height: 10,
fill: "blue",
stroke: null,
position: new go.Point(45, 40),
fromSpot: go.Spot.Bottom,
}),
$(go.Shape, "Circle", {
portId: "output2",
width: 10,
height: 10,
fill: "green",
stroke: null,
position: new go.Point(90, 20),
fromSpot: go.Spot.Right,
}),
$(go.Shape, "Circle", {
portId: "output3",
width: 10,
height: 10,
fill: "cyan",
stroke: null,
position: new go.Point(0, 20),
fromSpot: go.Spot.Left,
})
);
myDiagram.linkTemplate = $(
go.Link,
{ routing: go.Link.Orthogonal },
$(go.Shape),
$(go.Shape, { toArrow: "Standard" }),
$(go.TextBlock, "label", { segmentIndex: NaN, segmentFraction: 0.5 })
);
myDiagram.model = $(go.GraphLinksModel, {
linkFromPortIdProperty: "fromPort",
linkToPortIdProperty: "toPort",
nodeDataArray: [
{ key: "Alpha" },
{ key: "Beta" },
{ key: "Gamma" },
{ key: "Delta" },
],
linkDataArray: [
{ from: "Alpha", fromPort: "output", to: "Beta", toPort: "input" },
{ from: "Alpha", fromPort: "output2", to: "Gamma", toPort: "input" },
{ from: "Alpha", fromPort: "output3", to: "Delta", toPort: "input" },
],
});
</script>
</body>
</html>
Thank you so much for your time!