I think you could call Panel.findItemPanelForData and then call Panel.updateTargetBindings on the particular property that you are changing. That would be fairly efficient.
Here’s my test program. Note that unlike your situation I have made objA
references in two separate Panel.itemArrays within the same Node, as well as in different Nodes. GoJS does not naturally support objects shared between Parts, although it does within one Part. And for that matter, neither do Model.toJson and Model.fromJson, because they will lose the sharedness of the references.
function init() {
var $ = go.GraphObject.make;
myDiagram =
$(go.Diagram, "myDiagramDiv",
{
initialContentAlignment: go.Spot.Center, // for v1.*
"undoManager.isEnabled": true
});
myDiagram.nodeTemplate =
$(go.Node, "Vertical",
$(go.TextBlock, new go.Binding("text")),
$(go.Panel, "Auto",
$(go.Shape, { fill: "white" }, new go.Binding("fill", "color")),
$(go.Panel, "Horizontal",
$(go.Panel, "Vertical", { name: "FIRST", padding: 6 },
new go.Binding("itemArray", "items"),
{
itemTemplate:
$(go.Panel,
$(go.TextBlock,
new go.Binding("text"),
new go.Binding("stroke", "color"))
)
}),
$(go.Panel, "Vertical", { name: "SECOND", padding: 6 },
new go.Binding("itemArray", "items"),
{
itemTemplate:
$(go.Panel,
$(go.TextBlock,
new go.Binding("text"),
new go.Binding("stroke", "color"))
)
}),
)
)
);
var objA = { text: "objA" };
var objB = { text: "objB" };
var objC = { text: "objC", color: "red" };
myDiagram.model = new go.GraphLinksModel(
[
{ key: 1, text: "Alpha", color: "lightblue", items: [objA, objB, objC] },
{ key: 2, text: "Beta", color: "orange", items: [objA, objC] }
]);
window.toggleColor = function() {
myDiagram.commit(function(diag) {
diag.model.set(objA, "color", (objA.color === "green" ? "black" : "green"));
diag.nodes.each(function(n) {
var first = n.findObject("FIRST");
if (first) {
var ipan = first.findItemPanelForData(objA);
if (ipan) ipan.updateTargetBindings("color");
}
var second = n.findObject("SECOND");
if (second) {
var ipan = second.findItemPanelForData(objA);
if (ipan) ipan.updateTargetBindings("color");
}
});
}, "changed color");
};
}
Just call toggleColor
to change the stroke color of all TextBlocks that are bound to objA
's color
property.
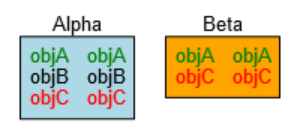