That row
variable is very suspicious. I’m surprised the code works reliably.
Here’s what I would do. In this screenshot the mouse is over or near the “2nd” text:
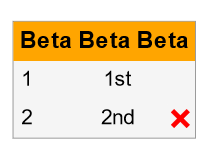
The complete code:
<!DOCTYPE html>
<html>
<head>
<title>Deleting Items</title>
<!-- Copyright 1998-2023 by Northwoods Software Corporation. -->
</head>
<body>
<div id="myDiagramDiv" style="border: solid 1px black; width:100%; height:400px"></div>
<textarea id="mySavedModel" style="width:100%;height:250px"></textarea>
<script src="https://unpkg.com/[email protected]"></script>
<script id="code">
const $ = go.GraphObject.make;
const myDiagram =
new go.Diagram("myDiagramDiv",
{
"undoManager.isEnabled": true,
"ModelChanged": e => { // just for demonstration purposes,
if (e.isTransactionFinished) { // show the model data in the page's TextArea
document.getElementById("mySavedModel").textContent = e.model.toJson();
}
}
});
myDiagram.nodeTemplate =
$(go.Node, "Auto",
$(go.Shape, { fill: null, stroke: "gray" }),
$(go.Panel, "Table",
{ background: "whitesmoke" },
$(go.Panel, "Auto",
{ row: 0, stretch: go.GraphObject.Fill },
new go.Binding("background", "color"),
$(go.TextBlock, { margin: 4, font: "bold 11pt sans-serif" },
new go.Binding("text"))
),
$(go.Panel, "Table",
{ row: 1, stretch: go.GraphObject.Fill },
new go.Binding("itemArray", "items"),
$(go.RowColumnDefinition, { column: 0, sizing: go.RowColumnDefinition.None }),
{
itemTemplate:
$(go.Panel, "TableRow",
{
mouseEnter: (e, pan) => pan.findObject("DeleteButton").visible = true,
mouseLeave: (e, pan) => pan.findObject("DeleteButton").visible = false,
background: "transparent" // capture mouse events for each TableRow Panel
},
$(go.TextBlock, { column: 0, margin: 5 },
new go.Binding("text", "id")),
$(go.TextBlock, { column: 1, margin: 5 },
new go.Binding("text", "t")),
$("Button", { column: 1, alignment: go.Spot.Right },
{
name: "DeleteButton",
visible: false, // default invisible
"ButtonBorder.strokeWidth": 0,
click: (e, button) => {
const elt = button.findBindingPanel();
const pan = elt.panel;
alert(`deleting item ${elt.data.id}: ${elt.data.t}`);
e.diagram.model.commit(m => {
m.removeArrayItem(pan.itemArray, elt.itemIndex);
});
}
},
$(go.Shape, "XLine", { width: 10, height: 10, stroke: "red", strokeWidth: 3 }))
)
}
)
)
);
myDiagram.model = new go.GraphLinksModel(
[
{ key: 1, text: "Alpha", color: "lightblue",
items: [
{ id: 1, t: "first" },
{ id: 2, t: "second" },
{ id: 3, t: "first" },
{ id: 4, t: "second" },
] },
{ key: 2, text: "Beta Beta Beta", color: "orange",
items: [
{ id: 1, t: "1st" },
{ id: 2, t: "2nd" },
] },
]);
</script>
</body>
</html>
Of course I’d remove the alert
call, at least.