I just tried modifying an old sample that had two “Table” Panels, and I added a mouseEnter event handler on the TextBlock in each “TableRow” Panel:
function init() {
var $ = go.GraphObject.make;
myDiagram =
$(go.Diagram, "myDiagramDiv",
{ "undoManager.isEnabled": true });
function collapsedArrow(shape) {
if (shape instanceof go.Shape) {
shape.figure = "TriangleRight";
shape.fill = "gray";
}
}
function expandedArrow(shape) {
if (shape instanceof go.Shape) {
shape.figure = "TriangleLeft";
shape.fill = "black";
}
}
var itemTemplate =
$(go.Panel, "TableRow",
$(go.TextBlock, { column: 0, minSize: new go.Size(50, NaN), maxSize: new go.Size(100, NaN) },
{ mouseEnter: function(e, tb) { console.log("item " + tb.panel.row) } },
new go.Binding("text", "desc")),
$(go.Shape, "TriangleRight",
{
name: "ARROW", column: 1,
width: 8, height: 8,
strokeWidth: 0, fill: "gray",
click: function(e, shape) {
e.handled = true;
e.diagram.startTransaction();
var details = shape.part.findObject("DETAILS");
if (shape.figure === "TriangleRight") {
// make sure all other Shapes are "TriangleRight" and gray
var items = shape.part.findObject("ITEMS");
if (items) items.elements.each(function(p) { collapsedArrow(p.findObject("ARROW")); });
// indicate this item is expanded, so show the details
expandedArrow(shape);
details.data = shape.panel.data;
details.visible = true;
} else {
collapsedArrow(shape);
details.visible = false;
}
e.diagram.commitTransaction("details");
}
})
);
var detailTemplate =
$(go.Panel, "TableRow",
$(go.TextBlock, { column: 0, minSize: new go.Size(50, NaN), maxSize: new go.Size(100, NaN) },
{ mouseEnter: function(e, tb) { console.log("detail " + tb.panel.row) } },
new go.Binding("text", "text")),
$(go.TextBlock, { column: 1 },
new go.Binding("text", "id"))
);
myDiagram.nodeTemplate =
$(go.Node, "Auto",
$(go.Shape, { fill: "white" }),
$(go.Panel, "Table", { margin: 2, defaultAlignment: go.Spot.Top },
$(go.Panel, "Table", { column: 0 },
new go.Binding("itemArray", "states"),
{ name: "ITEMS", itemTemplate: itemTemplate },
$(go.Panel, "TableRow", { row: 0, isPanelMain: true },
$(go.TextBlock, { column: 0, columnSpan: 2 },
new go.Binding("text", "key"))
)
),
$(go.RowColumnDefinition, { column: 1, width: 5 }),
$(go.RowColumnDefinition, { column: 2, separatorStroke: "black", separatorPadding: 5 }),
$(go.Panel, "Table", { column: 2 },
new go.Binding("itemArray", "details"),
{ name: "DETAILS", itemTemplate: detailTemplate, visible: false },
$(go.Panel, "TableRow", { row: 0, isPanelMain: true },
$(go.TextBlock, { column: 0, columnSpan: 2 },
new go.Binding("text", "desc"))
)
)
)
);
myDiagram.model = new go.GraphLinksModel([
{
key: "Alpha", states: [
{
desc: "A1", details: [
{ text: "info1a", id: "W" },
{ text: "info1b", id: "X" }
]
},
{
desc: "A2", details: [
{ text: "info2a", id: "W" },
{ text: "info2b", id: "X" },
{ text: "info2c", id: "Y" },
{ text: "info2d", id: "Z" }
]
},
{ desc: "A3", details: [] },
]
},
{
key: "Beta", states: [
{
desc: "U", details: [
{ text: "info1a", id: "U1" },
{ text: "info1b", id: "U2" }
]
}
]
}
],[
{ from: "Alpha", to: "Beta" }
]);
}
Everything seems to work as expected.
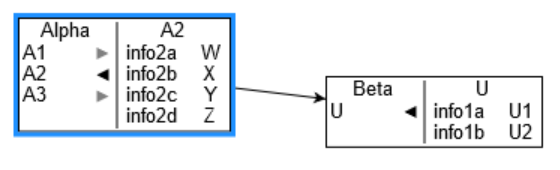