I have a table panel nested within a spot panel. The table panel has 3 rows: header, body and the footer. I want the body to resize and fill but the header and footer stay fixed in height and only grow in width.
But the moment I resize the node, the footer row disappears. See the gif attached. Here’s the code:
const mainTable = new go.Panel(go.Panel.Table, {
name: "box",
stretch: go.GraphObject.Fill,
minSize: new go.Size(240, 100),
alignment: go.Spot.TopLeft,
toLinkable: false,
})
// Column 0
.addRowColumnDefinition(
new go.RowColumnDefinition({
column: 0,
sizing: go.RowColumnDefinition.None,
})
)
// Column 1
.addRowColumnDefinition(
new go.RowColumnDefinition({
column: 1,
})
)
// Row 0
.addRowColumnDefinition(
new go.RowColumnDefinition({
row: 0,
sizing: go.RowColumnDefinition.None,
})
)
// Row 1
.addRowColumnDefinition(
new go.RowColumnDefinition({
row: 1,
sizing: go.RowColumnDefinition.Default,
stretch: go.GraphObject.Fill,
})
)
// Row 2
.addRowColumnDefinition(
new go.RowColumnDefinition({
row: 2,
sizing: go.RowColumnDefinition.None,
stretch: go.GraphObject.None,
})
)
.add(
new go.Picture({
source: "https://www.google.com/s2/favicons?sz=64&domain_url=figma.com",
width: 16,
height: 16,
row: 0,
column: 0,
alignment: go.Spot.MiddleLeft,
margin: 4,
})
)
.add(
// Header text
new go.TextBlock("Action 1", {
row: 0,
column: 1,
alignment: go.Spot.MiddleLeft,
margin: new go.Margin(0, 0, 0, 4),
verticalAlignment: go.Spot.MiddleLeft,
stretch: go.GraphObject.None,
editable: false,
}).bind(new go.Binding("text", "name").makeTwoWay())
)
.add(
// Description text
new go.TextBlock(
"Some description which are highly recommended in this day and age",
{
row: 1,
columnSpan: 2,
alignment: go.Spot.TopLeft,
stretch: go.GraphObject.Fill,
// margin: new go.Margin(8, 8, 8, 8),
background: "whitesmoke",
}
).bind(new go.Binding("text", "description").makeTwoWay())
)
.add(
new go.Panel(go.Panel.Auto, {
row: 2,
columnSpan: 2,
stretch: go.GraphObject.Fill,
background: "red",
}).add(
new go.Shape("Circle", {
fill: "orange",
strokeWidth: 0,
width: 16,
height: 16,
fromSpot: go.Spot.Center,
toSpot: go.Spot.Center,
cursor: "pointer",
})
)
);
const nodeTemplate = new go.Node(go.Panel.Spot, {
resizable: true,
resizeObjectName: "box",
background: "lightgray",
stretch: go.GraphObject.Fill,
})
.add(mainTable)
.add(
// Left
new go.Shape("Circle", {
fill: "gold",
width: 16,
height: 16,
alignment: go.Spot.LeftCenter,
alignmentFocus: go.Spot.Center,
})
)
.add(
// Right
new go.Shape("Circle", {
fill: "gold",
width: 16,
height: 16,
alignment: go.Spot.RightCenter,
alignmentFocus: go.Spot.Center,
})
)
.add(
// Top
new go.Shape("Circle", {
fill: "gold",
width: 16,
height: 16,
alignment: go.Spot.TopCenter,
alignmentFocus: go.Spot.Center,
})
)
.add(
// Bottom
new go.Shape("Circle", {
fill: "gold",
width: 16,
height: 16,
alignment: go.Spot.BottomCenter,
alignmentFocus: go.Spot.Center,
})
);
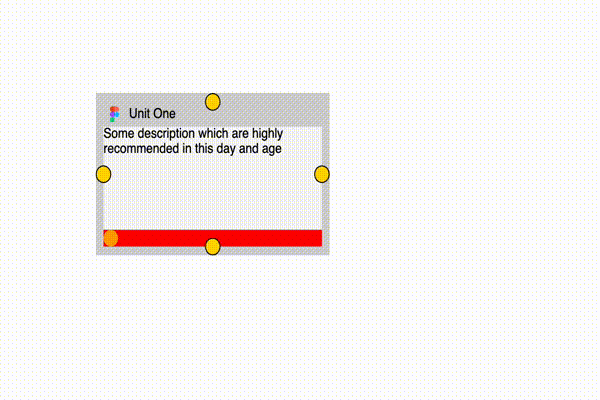